A program could contain run-time errors. The error can be logical or user-input error. These errors are considered as exceptions in C#. These exceptions are handled using the exception handler. If no exception handler is provided, the program may terminate without complete execution. This exception handling is derived from Sytem.Exception class. To define an exception, it should inherit from the ApplicationException class.
You can handle exceptions in C# in a program using the exception handler. These handlers are controlled structures. The exception is handled using three keywords: try, catch and finally.
Step 1: Implementation
Consider an example where some number is divided by zero. This throws a DivideByZeroException Exception. It is a runtime error. It occurs due to logical error. When this error is encountered, the default exception handler is invoked and it displays an error message.
This error can be tackled using an exception handler in C#. The try block is used to enclose the statements where the exception could be raised. The try block is followed by the catch block. It is a must to have one catch block at least, when talking about a try block. There can a number of catch blocks. When an exception is caught by the try block, an object of the exception is passed on to the catch block. The catch block that carries the respective exception as the parameter gets executed. The third element of the exception handler in C# is the finally block. The statements in the finally block gets executed even if there is no exception raised. The finally block is optional.
The following code shows the use of the try block, the catch block and the finally block:
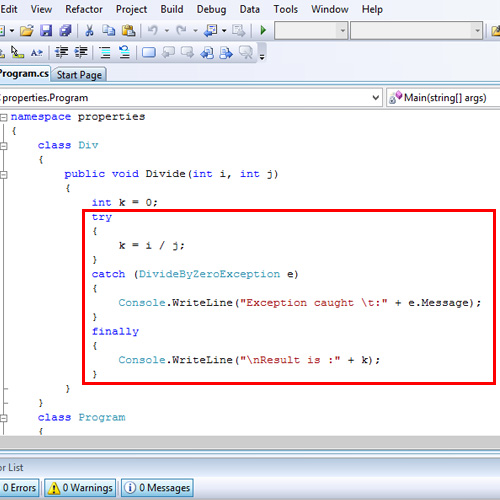
Step 2: Output
Here in the Main method, the class is initialized. The method Divide() is called. The parameters that are passed are 10 and 0, 0 in the denominator. During run-time, the compiler decides that 0 is not a divisor and throws an exception. The try block catches the exception and passes it to the catch block.
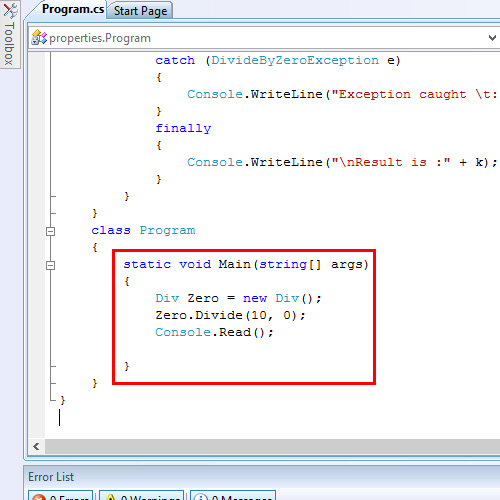
Step 3
Thus, the statements in the catch block get executed printing the error message followed by the statements in the finally block. Thus the exception does not force stop the program execution. The program terminates only after the complete execution of the code, as the exception is handled.
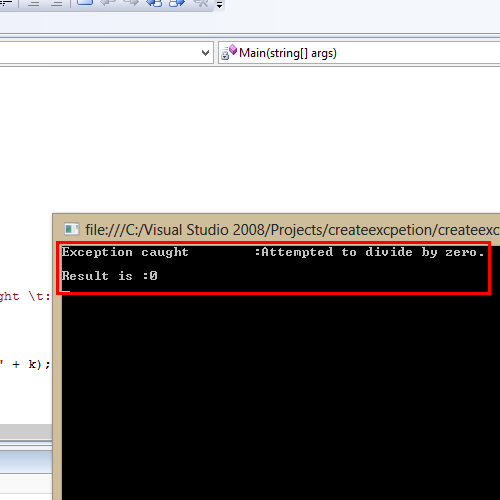