An event is an action, such as a mouse click or pressing a key. Events in C# are notifications sent by the object to indicate the occurrence of the event. These are messages sent to notify the change of state to the object.
In c#, delegates are used along with events for event handling. When an event is generated, the delegate calls the associated event handler. Consider an example of cricket, the event is hitting the ball with a bat. But depending on the position of the bat, the delegate will call the right event handler. If the position of the bat is right, then the delegate will choose the method associated with the ball moving left and if the position of the bat if left, then the delegate will choose the method associated will the ball moving right. Here the decision of the ball direction is taken when the ball hits the bat. When the event is generated, the delegate dynamically chooses the right method that handles the event.
Step 1: Implementation
Events in C# are of two parts: the subscriber and the publisher. A subscriber accepts the event and provides the handler for the event. A publisher contains the definition of the event and the delegate associated with it. Thus the delegate invokes the method. The delegate will be present in the subscriber class and the method in the publisher class. This method is the event handler. The subscriber class and the publisher class could be the same class.
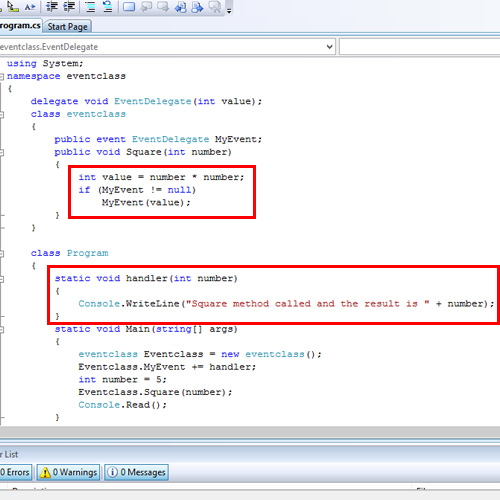
MyEvent() is the event raised whenever the Square() method is called. The delegate assigns the method to be called to the event, in this case the handler() method.
Step 2: Output
The compiler searches for the event handler during run-time. Thus the handler() method gets executed.
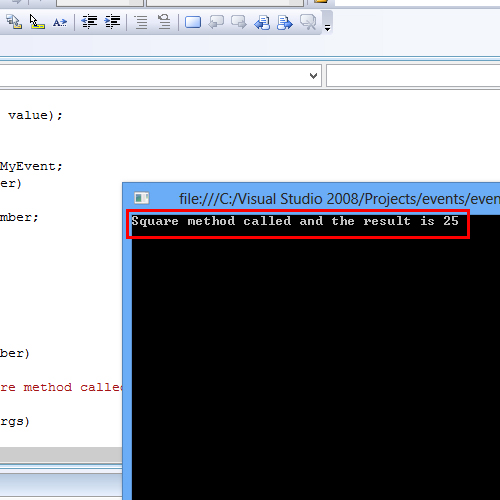
Note that, the if statement in the Square() method checks if the event has at least one subscribing delegate. The code throws an exception if there are no delegates. It is important that the handler() is invoked when the event is raised. This is ensured by the if, else construct that checks if MyEvent is not null.