Inheritance is an object oriented programming concept and is widely used to share commonly used members of a class with another class or multiple classes. A class in C# can inherit only from one class while a class can be inherited by unlimited classes.
The objects of the derived class get the copy of the data members and member functions of the base class by using inheritance. A class in C# that inherits from another class is called a derived or child class while the class from which attributes are derived is called a base or parent class.
Step 1: Implementing inheritance:
Each instance of a derived class includes its own members and all the members of the base class. Therefore the derived class has a large set of members. Inheritance avoids redundancy of code and enables easy maintenance. Any change made in the base class automatically reflects on the derived classes in C#.
The following syntax used to create derived class:
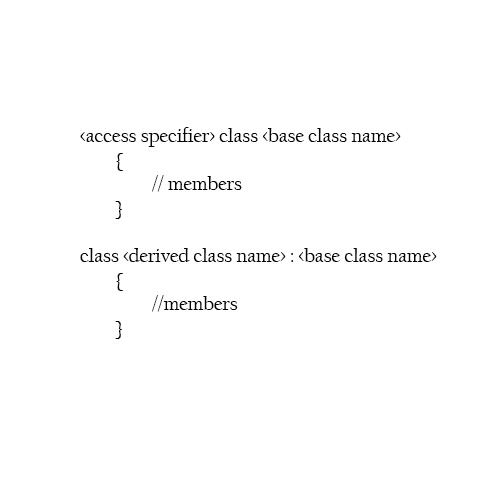
Step 2: Determining Inheritance Hierarchy:
Check the kind of relationship between the base class and the derived class. Ensure that the derived class is a kind of base class.
Consider an example of an Employee, Manager and Engineer classes. Here the Manager and Engineer class come under the employee class. The properties of the Employee class are required in the Manager and the Engineer classes.
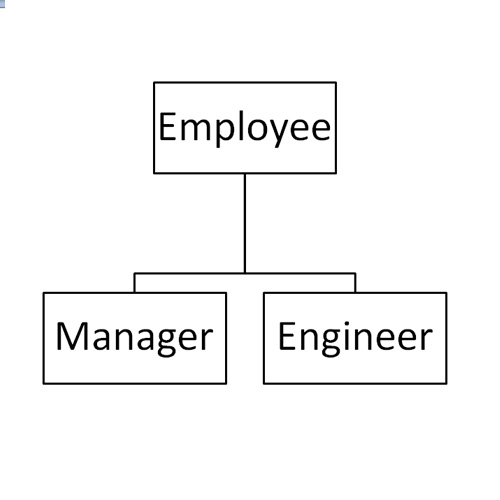
Step 3: Implementing Constructor:
To understand the implementation of class, let’s use constructors. A constructor us a special type of method that is invoked automatically when an instance of a class is created. The constructor must have the same name as the class. Consider the following program:
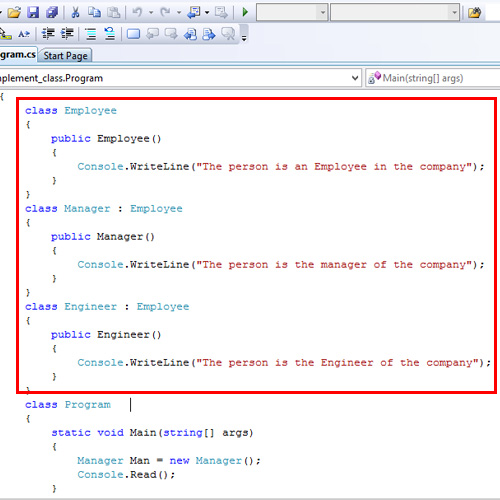
Step 4: Output
Here, the employee class is the base class. The manager and the engineer classes are the derived classes of the base class. When the derived class Manager is instantiated, the constructor of the base class in C# is called followed by the derived class constructor. The constructors can be used to initialize the member variables, if present in the classes in C#.
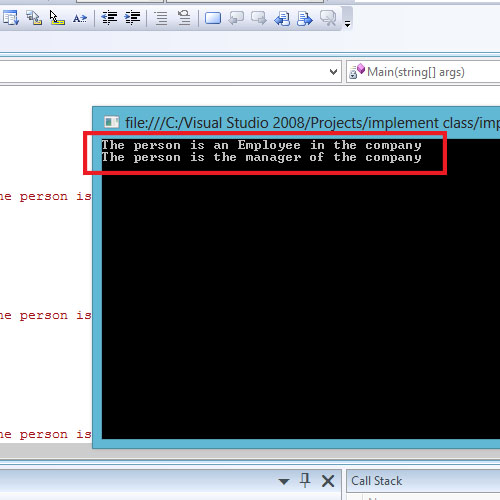