Polymorphism is a core behavior of object oriented programming. When implementing inheritance, the methods of the base class may have to be overridden. It is exactly for this purpose that the override modifier is provided. Thus method overriding in C# is implemented. The method in the base class should be marked as virtual.
The derived classes implement these virtual methods. Thus when overriding a method in C#, the ‘override’ keyword is used to specify the method that replaces its virtual base class method. This causes the base implementation to be ignored in favor of the “override” method.
Step 1: Implementation
The following code snippet shows the implementation of method overriding in c#. The method Designation() of the base class Employee is marked virtual to enable method overriding. The base class is derived by the class Manager. To override the method Designation() in the derived class, the method initialization contains the keyword ‘override’. Thus the method Designation() in the base class is hidden from the derived class.
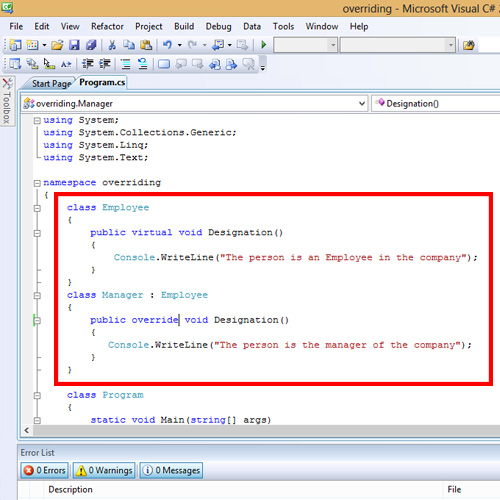
Step 2 – Override Keyword
The ‘override’ keyword is compulsory to denote the overriding method in C#. Otherwise, the method in base class is simply hidden by the method in the derived class. A warning will be generated if you compile the code after removing the override keyword.
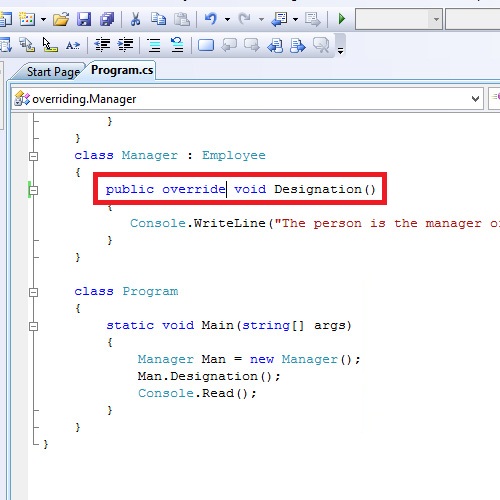
Step 3 – Output
When the method Designation() is called from the main method, the compiler calls the method that possess the ‘override’ keyword. Thus when the program is executed, the output will read “The person is the manager of the company”
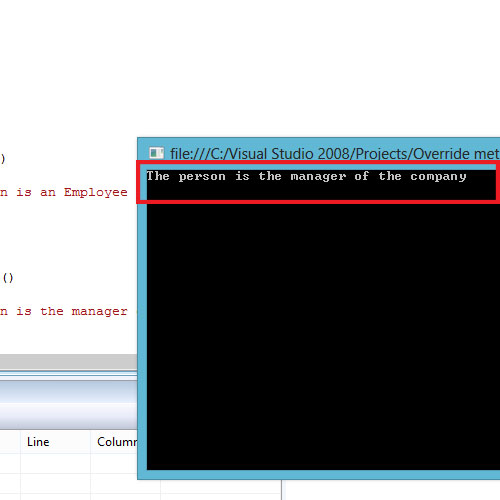