There will be times when you don’t need your class to be inherited by another class. You can restrict your class from being extended by using the ‘sealed’ keyword. The syntax is:
sealed class
{ //statements }
No class can be derived from a sealed class in C#. A sealed class cannot be declared abstract as well.
Similarly, a method can also be sealed to prevent it from overriding. Only methods with ‘override’ keyword can be sealed. When an override method is sealed, the base method is successfully overriden while this method cannot be overriden further.
Structs and properties can also be sealed. Structs are implicitly sealed and are not inherited.
Step 1: Implementation – Sealed class
The preceding code is an example of a sealed class in C#. When the sealed class Final is inherited by the class program, it results in an error. A sealed class cannot be inherited but the members of the sealed class can be accessed by instantiating the sealed class. Thus a sealed class is similar to any other class without inheritance.
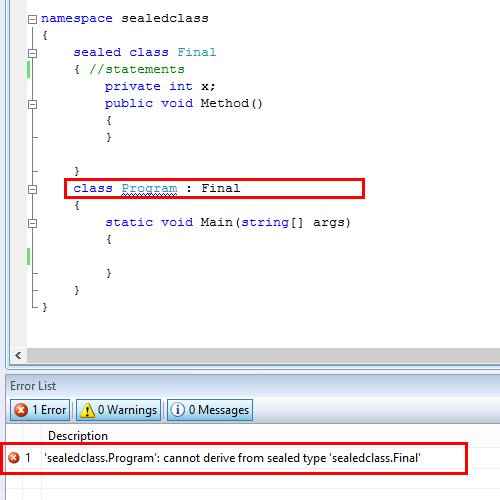
Step 2 – Implementation – Sealed methods
The usuage of a sealed method in C# is similar to any method. Note that a sealed method must be only used to override a method in the base class while the sealed method cannot be overriden by methods of another class. When a sealed class is overriden by the inherited class, it results in error. The class Third inherits from the class Second and the Method() is succesfully overriden and sealed. When this sealed Method() is overriden in the derived class Fourth, the compiler forces an error.
Thus the main advantage of using the sealed classes and sealed methods in C# is to take away the inheritance and define the boundary for the usage.
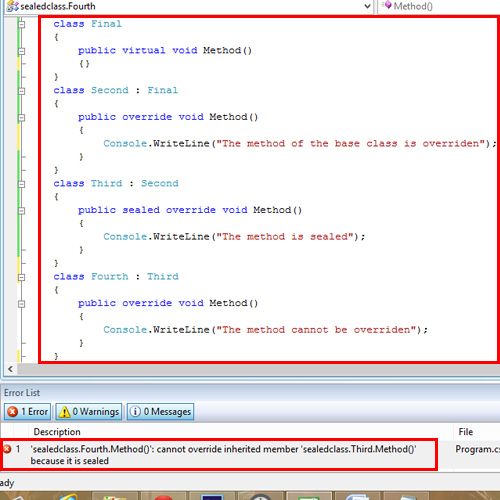