Parameters help in the flow of information to and from a method. When passing a parameter, they can be included inside the braces following the method name.
The syntax to declare a parameter is exactly like the one used to declare a variable. When more than one parameter is passed, they are separated using commas. The parameters are passed in braces in the method call.
The parameters are passed by value to a method. This is referred to as variable parameters in C#. New memory locations are created for all the parameters in the method call. The values of the parameters are copied in those locations. So the changes in the value made inside the method does not reflect on the parameters in the method call.
You should make sure that the data type of theparameters being passed should match the parameters’ data type specified in the method declaration.
Step 1: Implementation – Variable parameter
In the following code snippet, the variable Variable within the square() method is of integer data type and the method call contains the variable number inside the square() method is also of integer data type:
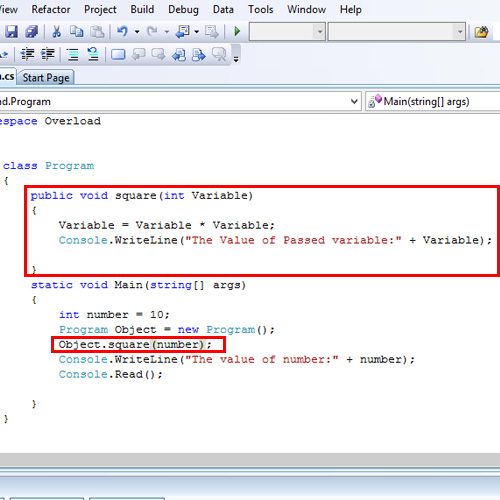
Step 2 –The Variable Parameter
When you run the program you can note that as the value of Variable changes, the value of the number does not change. They both have different memory allocation.
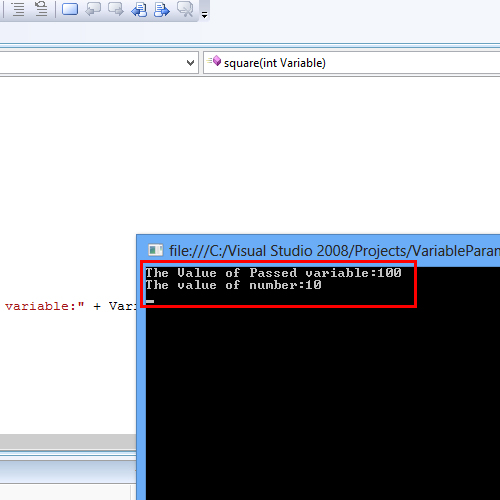
Step 3: Implementation – Return type
As values flow into the method, it is also possible to get values from the method. This is done using the return variable in C#. The ‘return’ keyword can return one value to the method call. The ‘void’ keyword in the method declaration should be changed to the data type of the value being returned. Thus the method in the previous example:
public void square(int Variable){}
is replaced as:
public int square(int Variable){}
Notice the the ‘void’ keyword is replaced by int.
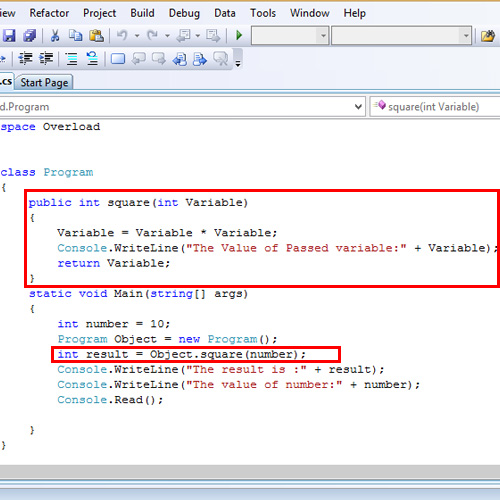
Step 4 – The return variable
The function call returns a value. So a variable should be initialized to receive the value. The value is returned to the method call by the statement return Variable;
Thus the variable result holds the square of the number.
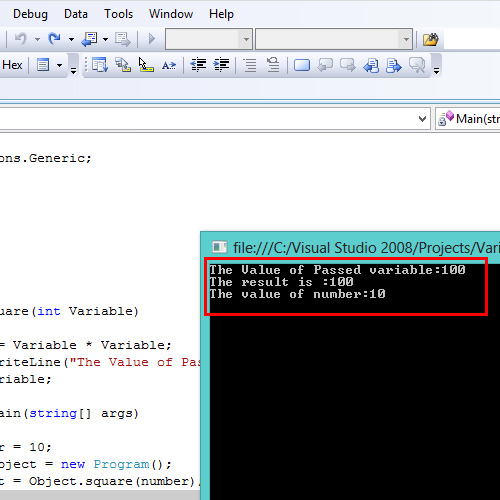