Delegates in C# are used to refer a method. The compiler dynamically chooses the method in a class depending on the delegate. This gives flexibility in programming.
A delegate needs to be declares, instantiated and used. Note that a delegate can refer to only those methods that hold the same signature. Delegate is also a keyword used and the syntax is as follow:
<access modifier> delegate <return type> <delegatename&ggt;(<Parameters>);
Step 1: Implementation
A C# delegate is declared prior to the method in which it is used. The declaration of the delegate should possess the signature of the method to be referenced. The delegate is then instantiated in the Main method. The method to be called when the delegate is invoked is passed as a parameter in the delegate initialization. The delegate call is similar to the method call
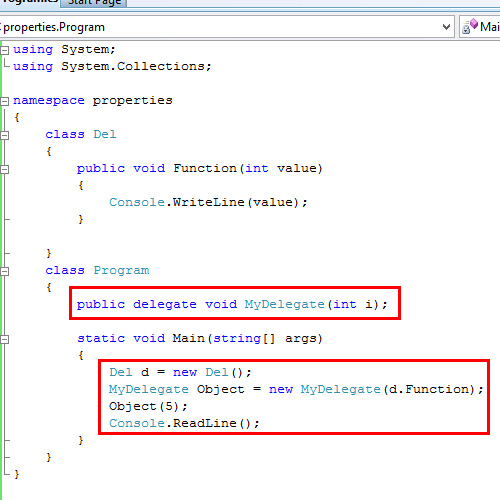
Step2: Output
Thus when the program is executed, the compiler locates the method that is referenced by the Object of delegate in C#. The method d.Function() is called and the parameter 5 is passed to the method. Thus, this value gets printed on to the screen
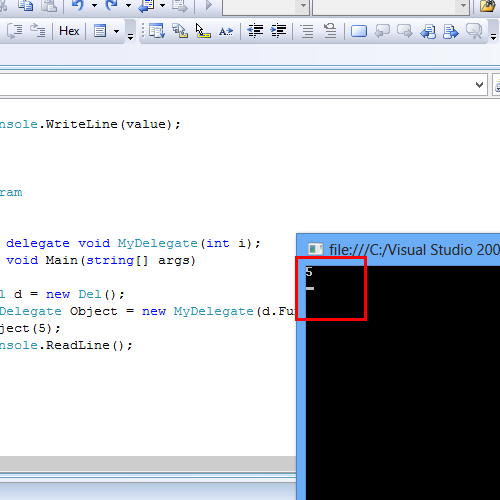
Step 3: Implementing Multicast delegate
The delegates are of two types:
• Single-cast delegate
• Multicast delegate
The previous example is a single-cast delegate where the delegate is referenced to only one method. A multicast delegate refers to multiple methods at a time. The += operator is used to add methods to the delegate. The following example shows how to add multiple methods to a delegate.
Multicast delegate in C# is present in the class, System.MulticastDelegate. The following program contains three methods that are referenced by a single delegate:
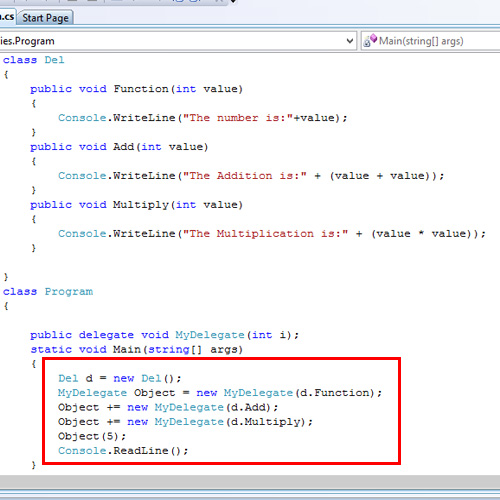
Step 4: Output for Multicase delegate
The multicast delegate executes the methods in the order they are added to the delegate. It is compulsory to ensure that the methods possess the same signature as that of the delegate. Using the same delegate, you can call Add(), Multiply() and Function() methods in one call. The output is as follows:
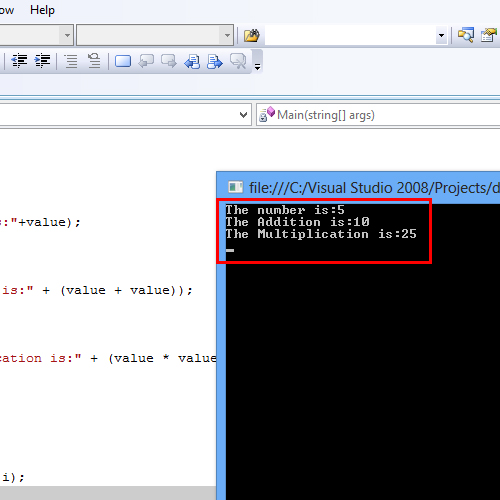
Step 5 – Removing a method
An assigned method can be removed from a delegate using the -= operator.
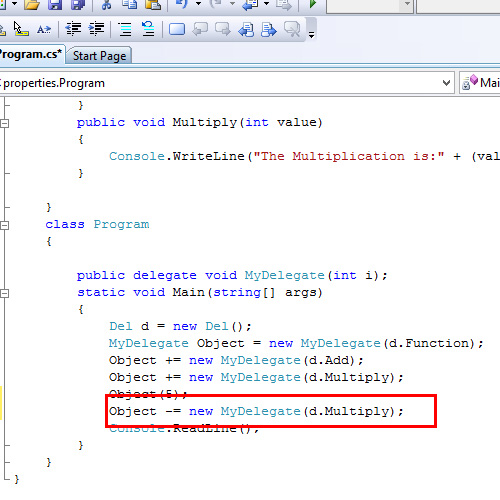