Interfaces in C# are similar to classes with no implementation. They are the template on the members the class should possess. Thus interface contains the member declarations. Classes and structures implement these members. Interface is the standard template you want to implement on the classes that inherit interface. A class can inherit multiple interfaces. Interfaces implement runtime polymorphism.
The concept behind the inheritance of the interface in C# is called name hiding. It is the ability to hide the name of the members outside the derived class.
You need to declare and implement an interface. You can declare methods and properties in an interface. You cannot declare variables in an interface. The syntax is as follows:
public interface <interfacename>
{
//members
}
You can declare an interface in C# using the ‘interface’ keyword. The interface contains methods that need to be implemented by the class that inherits this interface. Interface can inherit from an existing interface. The class, which implements the interface, must implement all the members of the interface.
Step 1: Implementation
Consider the following code snippet. It is a two level inheritance. In the first level, the code shows the inheritance of an interface from an existing interface. In the second level, a class inherits the interface. The class that inherits the interface must also implement the members of the base interface. Thus in the preceding code, both ParentMethod() and ImplementMethod() are implemented in the MyClass.
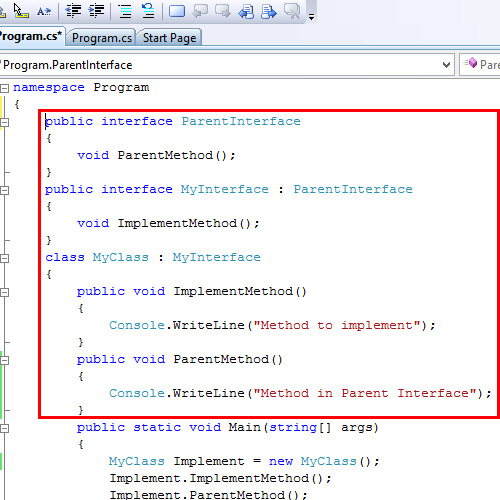
Step 2: Output:
In the main method, the class is initialized and the member methods are called. When executed, the statements in both the method get printed. The following is the output:
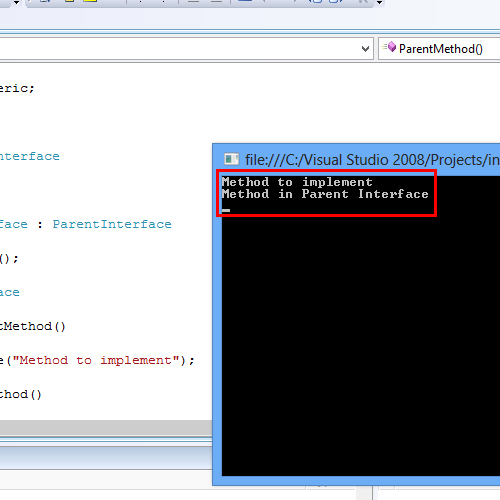
Step 3 – Error while implementation
When implementing an interface in C#, note that all the members are implemented. Here the members are ImplementMethod() and ParentMethod(). Both should be implemented. If any one of the member is missing in the implementation, the compiler posts an error. Thus when ParentMethod() is commented, an error is raised as the following:
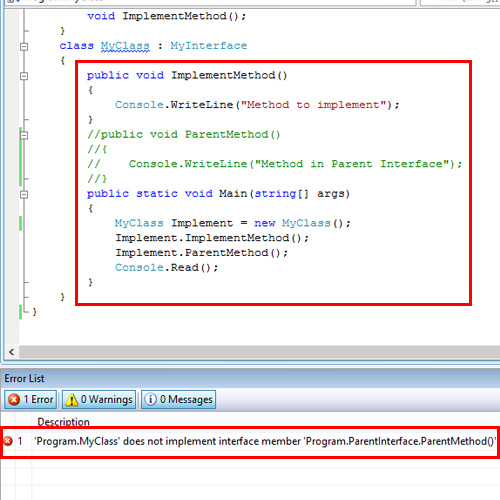