Abstract class In Object Oriented Programming (OOP) is a type of class which is incomplete within itself, but they are created and used for inheriting (deriving) a sub class (derived class).
To create c# abstract classes following pattern is followed:
Declare a class as ‘abstract’ before the class definition, e.g
Public abstract class Animal
{
//Here goes class attributes and members
}
The abstract class can contain methods but these methods are again Abstract i.e., their functionality is not defined in the abstract class but they are used and defined in the derived class.
For creating abstract methods in c#, we just put a keyword abstract before method name
For Example:
Public abstract class demo
{
Public abstract void Sound ();
}
Here, Sound is actually an abstract method which does not show its functionality in this class but its functionality would be defined in the derived class in which it has to be used.
An important point must be noticed that a semicolon (;) must be provided after declaring an abstract method which is an unusual type of declaration than normal methods, but in the case of abstract methods, it has to be followed.
Step 1- Implementation and Result
The following code shows the use of c# abstract classes and methods.
using System;
using System.Collections;
namespace properties
{
abstractclassAgeGroup
{
Public abstract void Activity();
}
classchild : AgeGroup
{
Public over ride void Activity()
{
Console.WriteLine(“Play time”);
}
}
classAdult : AgeGroup
{
publicoverridevoid Activity()
{
Console.WriteLine(“Nap time”);
}
}
classProgram
{
staticvoid Main(string[] args)
{
child MyChild = newchild();
Adult MyAdult = newAdult();
Console.Write(“Time for Child :”);
MyChild.Activity();
Console.Write(“Time for Adult :”);
MyAdult.Activity();
Console.Read();
}
}
}
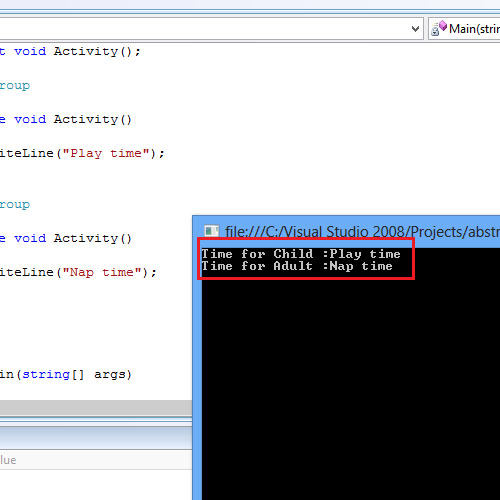
And this was how we can create abstract methods in c# along with their classes.