Hash table is basically a data structure which holds some information along with its reference number. Hash table is implemented in an array which works in such a way that the information is stored inside the array in the index number provided. Thus, it can be retrieved or accessed directly with the help of the index number instead of searching through the array.
In this article, we will teach you to create Java hash table
Step 1 –Import Array Library & define a function
In order to do so, you will need a class. In our case, it is “Hash Function”.
In order to use the arrays and their functions, we will have to import the array library which goes by the name java.util.arrays.
Inside the class, create a string array, declare a variable for array size and then declare a variable for items in array as zero.
Now, you will have to define some functions for Java hash table.
Start a function as “Hash Function” outside the main. This function will receive a size as an argument.
It will create a string array depending on that size and will then be responsible to fill that array.
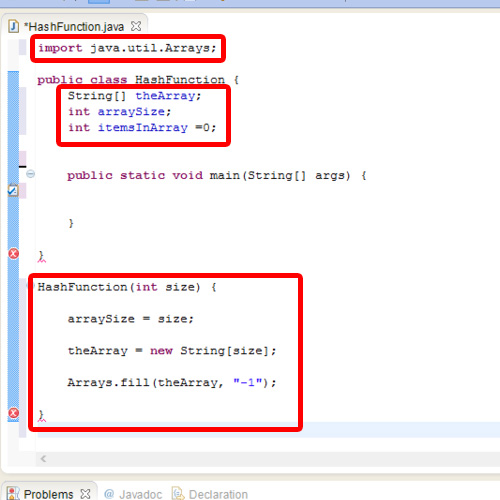
Step 2 – Function to Add Values
After that, create a function to display the Java hashtable. This function will simply print the data in a table format. That’s why it contains formatting and alignment techniques.
Next, we will create another method and name it as “hash function 1”.
This function will actually put the value provided for the array in the same index that matches that value. This function will receive numbers for array so we have specified the array type as string in the parameter. It will store these numbers in our array called “the array”.
Inside the function body, we have started a loop to fill the array with the values. In the last line, we are converting the string to integer as it will make it easier to perform calculations. The new element val keyword is responsible to validate the duplication i.e. it will ensure that the values are not duplicates.
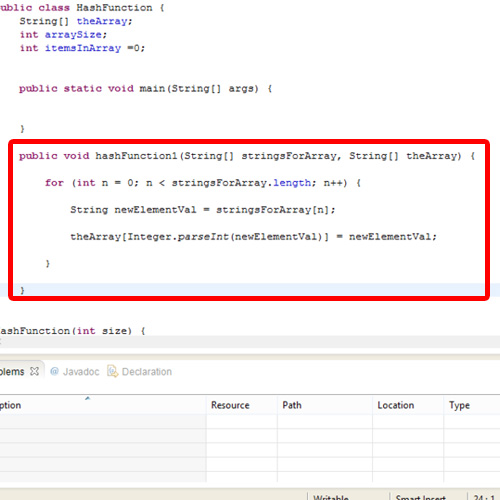
Step 3 – The Main body
Now let us consider the main body. Over here, we have created an object of our class as “the func”. We have defined 30 in the argument which means that our array would be 30 in length. Right below, we have specified the numbers to be inserted in the array. After that, we are just calling our function to insert the values and then later, we are calling the display function.
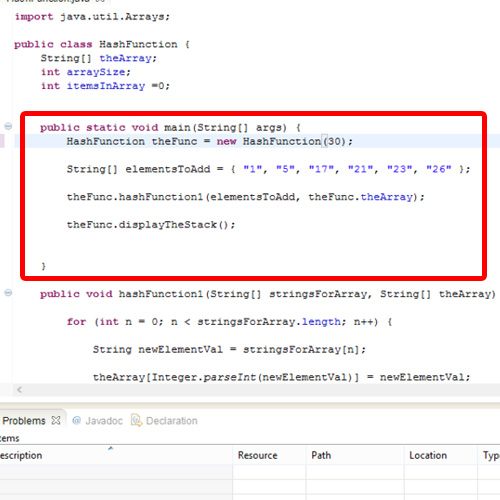
Step 4 – View table output
Now let us execute this. You will notice that it has made an array of thirty locations and has placed the values on the same index number which matches its value number.
In this manner, we can create a simple Java hashtable.
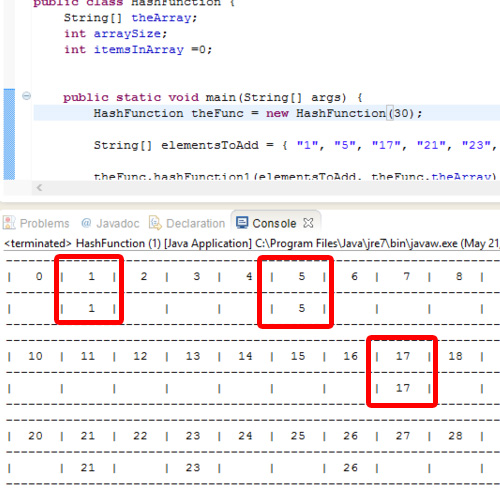